缸鸭狗&牛油果
IOT PROJECT
this project use Node MCU hard board and Ali cloud service to control the move of a servo .
operation process
1. Materials Need
1. a USB cable
2. a Node MCU hard board
3. a serve
4. 3 electric wires
5. Ali cloud service
2. Operation
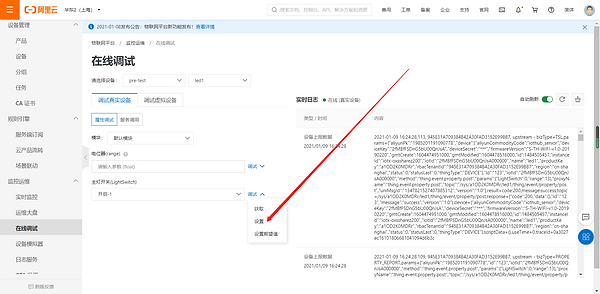
1. open the switch of servo in Ali IOT platform
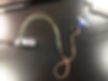
2. servo move

3. monitor record servo move
3. Code
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
#include <ArduinoJson.h>
#include <aliyun_mqtt.h>
#define SENSOR_PIN 13 //pin define
#define LED D4
#define LED2 D5
#define PRODUCT_KEY "a1OD2K0MDRr"//exchange PRODUCT_KEY
#define DEVICE_NAME "led1"//exchange DEVICE_NAME
#define DEVICE_SECRET "d6b122352255884bddbb6f249401c6f9"//exchange DEVICE_SECRET
#define DEV_VERSION "S-TH-WIFI-v1.0-20190220"
#define WIFI_SSID "幻想工程师"//exchange WIFI
#define WIFI_PASSWD "111111112"//exchange WIFI password
//构建舵机
#include <Servo.h>
Servo servo; // create servo object to control a servo
int pos = 90; // variable to store the servo position min:45 max:135
#define servoAttach D6 //舵机针脚9
#define ALINK_BODY_FORMAT "{\"id\":\"123\",\"version\":\"1.0\",\"method\":\"%s\",\"params\":%s}"
#define ALINK_TOPIC_PROP_POST "/sys/" PRODUCT_KEY "/" DEVICE_NAME "/thing/event/property/post"
#define ALINK_TOPIC_PROP_POSTRSP "/sys/" PRODUCT_KEY "/" DEVICE_NAME "/thing/event/property/post_reply"
#define ALINK_TOPIC_PROP_SET "/sys/" PRODUCT_KEY "/" DEVICE_NAME "/thing/service/property/set"
#define ALINK_METHOD_PROP_POST "thing.event.property.post"
#define ALINK_TOPIC_DEV_INFO "/ota/device/inform/" PRODUCT_KEY "/" DEVICE_NAME ""
#define ALINK_VERSION_FROMA "{\"id\": 123,\"params\": {\"version\": \"%s\"}}"
unsigned long lastMs = 0;
int val=0;
WiFiClient espClient;
PubSubClient mqttClient(espClient);
void init_wifi(const char *ssid, const char *password)
{
WiFi.mode(WIFI_STA);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED)
{
Serial.println("WiFi does not connect, try again ...");
delay(500);
}
Serial.println("Wifi is connected.");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void mqtt_callback(char *topic, byte *payload, unsigned int length)
{
Serial.print("Message arrived [");
Serial.print(topic);
Serial.print("] ");
payload[length] = '\0';
Serial.println((char *)payload);
Serial.println("");
Serial.println((char *)payload);
Serial.println("");
if (strstr(topic, ALINK_TOPIC_PROP_SET))
{
StaticJsonBuffer<100> jsonBuffer;
JsonObject &root = jsonBuffer.parseObject(payload);
int params_LightSwitch = root["params"]["LightSwitch"];
if(params_LightSwitch==1)
{ Serial.println("servo work");
digitalWrite(LED,HIGH);
for (pos = 90; pos <= 135; pos += 1) { // goes from 0 degrees to 180 degrees
servo.write(pos); // tell servo to go to position in variable 'pos'
delay(5); // waits 15ms for the servo to reach the position
}
for (pos = 135; pos >= 45; pos -= 1) { // goes from 180 degrees to 0 degrees
servo.write(pos); // tell servo to go to position in variable 'pos'
delay(5); // waits 15ms for the servo to reach the position
}
for(pos = 45 ; pos <= 90 ; pos += 1){
servo.write(pos); // tell servo to go to position in variable 'pos'
delay(5);
}
}
else
{ //Serial.println("Led off");
digitalWrite(LED,LOW);}
if (!root.success())
{
Serial.println("parseObject() failed");
return;
}
}
}
void mqtt_version_post()
{
char param[512];
char jsonBuf[1024];
//sprintf(param, "{\"MotionAlarmState\":%d}", digitalRead(13));
sprintf(param, "{\"id\": 123,\"params\": {\"version\": \"%s\"}}", DEV_VERSION);
// sprintf(jsonBuf, ALINK_BODY_FORMAT, ALINK_METHOD_PROP_POST, param);
Serial.println(param);
mqttClient.publish(ALINK_TOPIC_DEV_INFO, param);
}
void mqtt_check_connect()
{
while (!mqttClient.connected())//mqtt���
{
while (connect_aliyun_mqtt(mqttClient, PRODUCT_KEY, DEVICE_NAME, DEVICE_SECRET))
{
Serial.println("MQTT connect succeed!");
//client.subscribe(ALINK_TOPIC_PROP_POSTRSP);
mqttClient.subscribe(ALINK_TOPIC_PROP_SET);
Serial.println("subscribe done");
mqtt_version_post();
}
}
}
void mqtt_interval_post()
{
char param[512];
char jsonBuf[1024];
//sprintf(param, "{\"MotionAlarmState\":%d}", digitalRead(13));
sprintf(param, "{\"LightSwitch\":%d,\"range\":%d}",!digitalRead(LED),val);
sprintf(jsonBuf, ALINK_BODY_FORMAT, ALINK_METHOD_PROP_POST, param);
Serial.println(jsonBuf);
mqttClient.publish(ALINK_TOPIC_PROP_POST, jsonBuf);
}
void setup()
{
pinMode(SENSOR_PIN, INPUT);
pinMode(LED, OUTPUT);
pinMode(LED2, OUTPUT);
/* initialize serial for debugging */
Serial.begin(115200);
Serial.println("Demo Start");
init_wifi(WIFI_SSID, WIFI_PASSWD);
mqttClient.setCallback(mqtt_callback);
servo.attach(D6);
servo.write(0); //设置舵机初始角度为0
}
// the loop function runs over and over again forever
void loop()
{
val= analogRead(A0);
if (val>500)
{
digitalWrite(LED2,HIGH);
}
else
{digitalWrite(LED2,LOW);}
if (millis() - lastMs >= 5000)
{
lastMs = millis();
mqtt_check_connect();
/* Post */
mqtt_interval_post();
}
mqttClient.loop();
unsigned int WAIT_MS = 2000;
if (digitalRead(SENSOR_PIN) == HIGH)
{
Serial.println("Motion detected!");
}
else
{
Serial.println("Motion absent!");
}
delay(WAIT_MS); // ms
Serial.println(millis() / WAIT_MS);
}